CupertinoActionSheetAction
An action button typically used in CupertinoActionSheet
.
Examples
Basic Example
- Python
import flet as ft
def main(page):
page.horizontal_alignment = ft.CrossAxisAlignment.CENTER
def handle_click(e):
page.add(ft.Text(f"Action clicked: {e.control.content.value}"))
page.close(bottom_sheet)
action_sheet = ft.CupertinoActionSheet(
title=ft.Row([ft.Text("Title")], alignment=ft.MainAxisAlignment.CENTER),
message=ft.Row([ft.Text("Description")], alignment=ft.MainAxisAlignment.CENTER),
cancel=ft.CupertinoActionSheetAction(
content=ft.Text("Cancel"),
on_click=handle_click,
),
actions=[
ft.CupertinoActionSheetAction(
content=ft.Text("Default Action"),
is_default_action=True,
on_click=handle_click,
),
ft.CupertinoActionSheetAction(
content=ft.Text("Normal Action"),
on_click=handle_click,
),
ft.CupertinoActionSheetAction(
content=ft.Text("Destructive Action"),
is_destructive_action=True,
on_click=handle_click,
),
],
)
bottom_sheet = ft.CupertinoBottomSheet(action_sheet)
page.add(
ft.CupertinoFilledButton(
"Open CupertinoBottomSheet",
on_click=lambda e: page.open(bottom_sheet),
)
)
ft.app(main)
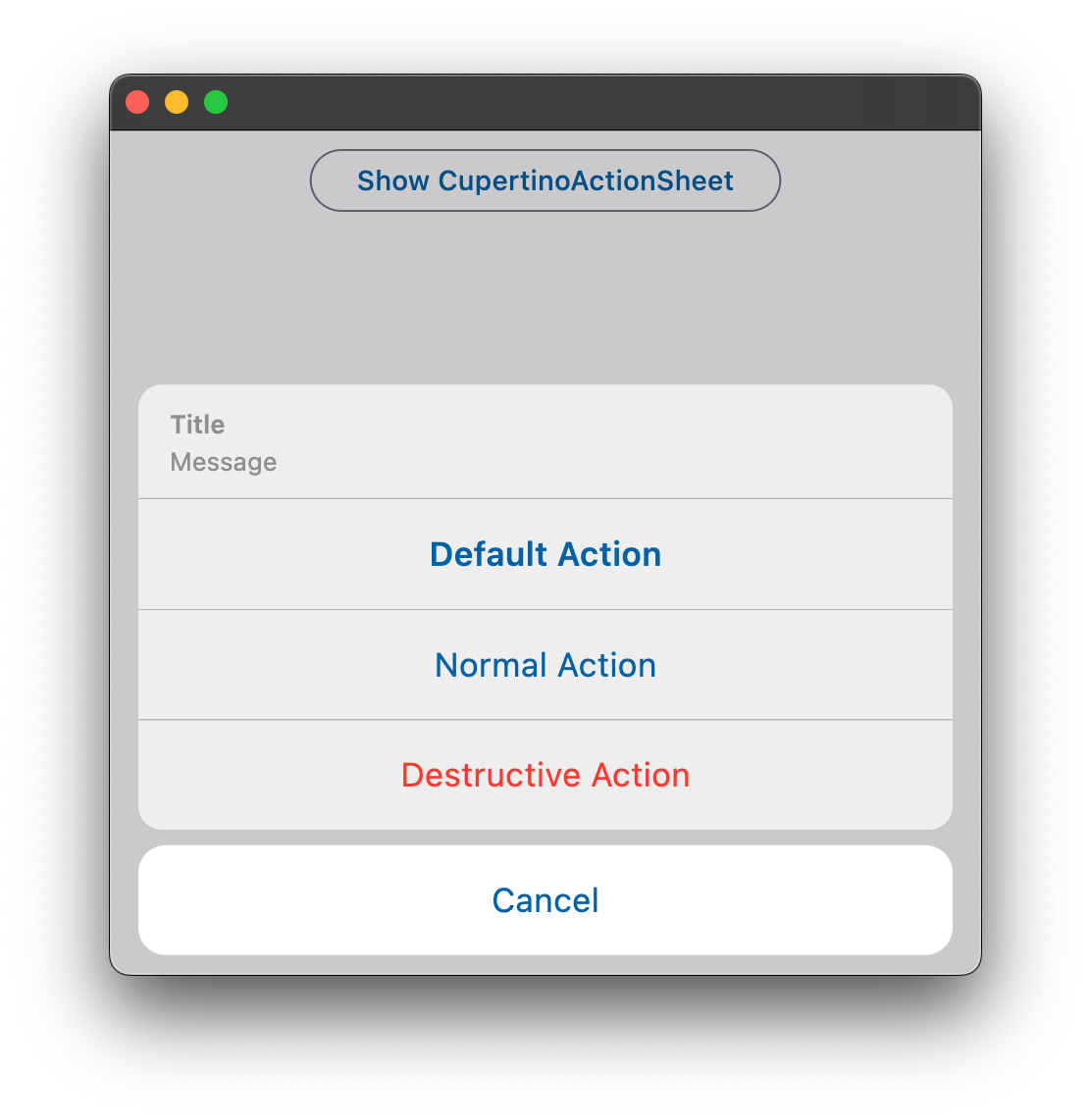
Properties
content
The child control to be shown in this action button. In case both text
and content
are provided, then content
will
be used.
is_default_action
Whether this action should receive the style of an emphasized, default action.
Defaults to False
.
is_destructive_action
Whether this action should receive the style of a destructive action.
Defaults to False
.
text
The text to be shown in the button. In case both text
and content
are provided, then content
will be used.
Events
on_click
Fires when this action button is clicked.